How to fix error - Property does not exist on type DefaultRootState
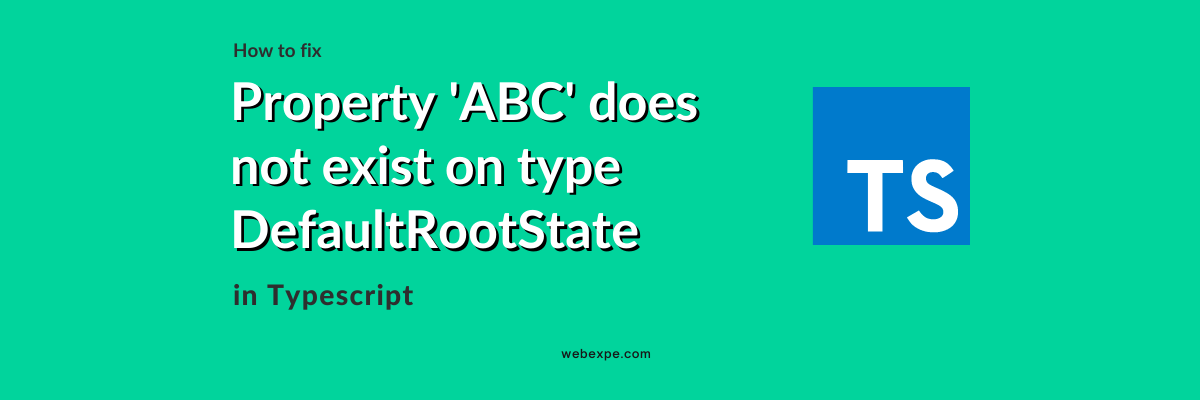
One error that TypeScript developers frequently run into is
"Property 'ABC' does not exist on type 'DefaultRootState'"
Lets see why this error occurs and how to fix it.
Why "Property 'ABC' does not exist on type 'DefaultRootState'" occurs ?
This error occurs when TypeScript cannot find the property within the 'DefaultRootState' type.
Let's understand this using an example
Consider we have a redux store and a reducer created to store userDetails as shown below:
// userDetails.ts interface Iinitialstate { users: Array<object>; isLoggedIn: boolean; } const initialstate : Iinitialstate = { users: [], isLoggedIn: false } const userDetailsReducer = (state = initialstate, action: Action) => { switch (action.type){ case "updateUserData": { return { // some logic } } default: return state; } } export default userDetailsReducer;
And we are trying to read the state from reducer in our component User.ts as shown below
// Users.ts import { useSelector } from 'react-redux'; const Users = () => { const users = useSelector((state) => state?.userDetails); }
But now when we run this code we will get this error - "Property 'userDetails' does not exist on type 'DefaultRootState'"
How to fix the "Property 'userDetails' does not exist on type 'DefaultRootState'" error ?
-
To resolve the error, we need to import RootStateOrAny type provided by react-redux library. The RootStateOrAny type allows us to access the Redux store state without specifying the exact type.
-
Assign RootStateOrAny as the type for the state parameter in the useSelector hook, so that we can access the userDetails property from the Redux store as shown below.
// Users.ts import { useSelector, RootStateOrAny } from 'react-redux'; const Users = () => { const users = useSelector((state: RootStateOrAny) => state?.userDetails); }
Conclusion
This solution can also be helpful in scenarios where the exact type of the state is unknown or when dealing with complex store structures.