How to create Custom Hooks in ReactJS | React interview question.
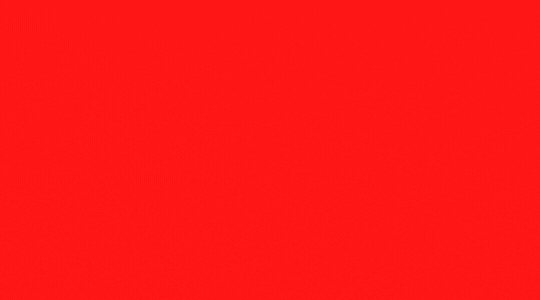
In this article we are going to have a look at how to create our own hooks. So let's dive in.
What is a custom hook?
let's take a look at the defination at reactjs.org
Custom Hooks are a mechanism to reuse stateful logic Example: setting up a subscription and remembering the current value, but every time you use a custom Hook, all state and effects inside of it are fully isolated.
Custom hooks are a powerful feature in React, and if you are not using them in your React applications, you are missing out on a lot of benefits.
When should one write a custom hook in react?
when you have a common logic written for a state's update in more than one component, we can turn it into a custom hook.
Benefits of Using Custom Hooks
- Reduces redundant code.
- Every time you call a hook all your state and effects inside of it are fully isolated that means you can call the same hook twice in one
component.
Rules to write a custom hook
- Prerequisite : use React version 16.8. and above
- Custom hook name starts with "use". This convention is very important. As React would be able to automatically check for violations of rules of Hooks incase of error because a normal function call does not convey if a certain function contains calls to Hooks inside of it.
How to Create Custom Hooks
Now that we have a basic understanding of a custom hook, let's get started and create a custom hook to use a loader in a component to handle asynchronous operations.
Loaders are a basic necessity in every website that are shown on the screen to signal the user that some data is being asynchronously fetched.
Here is a step by step guide:
- Create a folder named hooks to keep all the hooks at one place.
- Inside it create a UseLoader.js file.
- As shown in the code snippet below we have used useState to create a state to show/hide the loader.
// inside /hooks/UseLoader.js import { useState } from "react"; const UseLoader = ({ isLoading = false }) => { // resusable stateful logic const [isLoading, setLoading] = useState(isLoading); return [isLoading, setLoading]; } export default UseLoader;
How to Use Custom Hook in react component
Using a custom hook in React is similar to using any other hook. You simply call the hook function and pass in any necessary parameters.
-
First we import the hook at the top of our file.
// inside /components/Home/index.js import Loader from '../loader'; import useLoader from '../../hooks/useLoader'; // importing the hook
-
call useLoader and set its default value as false. useLoader gives us two things- a variable to read the state and a setter to update the loader state.
// setting isLoading as false initially const [isLoading, setLoading] = useLoader(false);
-
The loader is then set to true immediately before the api call inside useEffect and is changed back to false once we receive a response or encounter an error.
// setting isLoading as false initially const [isLoading, setLoading] = useLoader(false); useEffect(() => { const getCustomerDetails = async () => { // setting the loader as true setLoading(true); try { await fetch(custApiUrl); // setting it as false after the api call setLoading(false); } catch (e) { // setting it as false incase of errors setLoading(false); } }; getCustomerDetails }, []);
-
The final home component file should look something like this:
// inside /components/Home/index.js import Loader from '../loader'; import useLoader from '../../hooks/useLoader'; // importing the hook const Home = () => { // setting isLoading as false initially const [isLoading, setLoading] = useLoader(false); useEffect(() => { const getCustomerDetails = async () => { // setting the loader as true setLoading(true); try { await fetch(custApiUrl); // setting it as false after the api call setLoading(false); } catch (e) { // setting it as false incase of errors setLoading(false); } }; getCustomerDetails }, []); return ( <> {isLoading && <Loader />} {!isLoading && ( <div> ... some jsx to show customer details </div> )} </> ); } export default Home;
And that's it we have successfully created a custom hook and also saw how easy it is to use in components.
Conclusion
In conclusion, custom hooks are a powerful feature in React that can help you write cleaner, more reusable code. By extracting logic into separate hooks, you can focus on what your component does, rather than how it does it.