What is Context API and useContext Hook in React? | React Interview Question
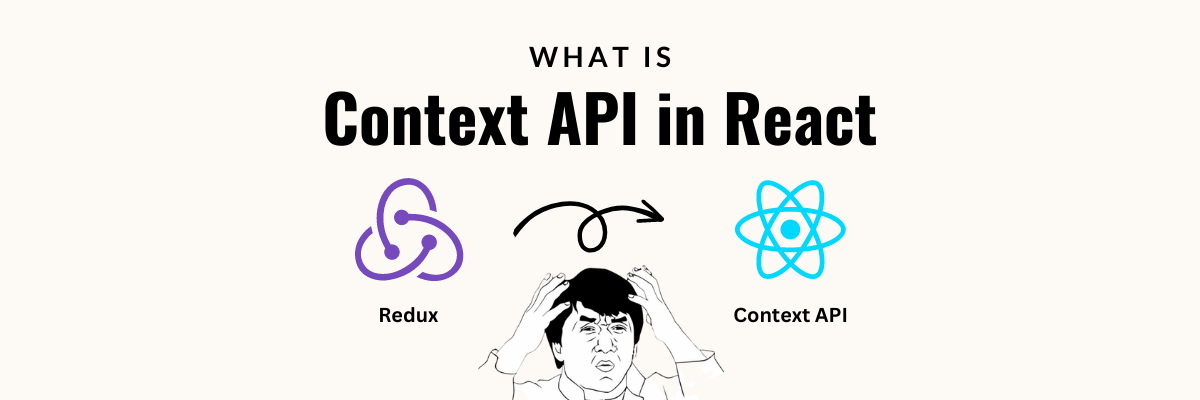
React context API was released in React version 16.3 and was appreciated from day one of the release as it served as a global state across components for small to medium sized applications.
In this article, we will see what is Context API and when and how to use it. So let's get started!
what is Context API?
The Context API in React provides a way to pass data through the component tree without the need to pass props down manually at every level. The global state created using context API can be accessed by any component within the tree, regardless of how close they are to one another in the hierarchy.
When to use Context API?
- To eliminate prop drilling
Using Context API eliminates the need for prop drilling, where props are passed from a higher-level component to a lower-level component through multiple layers.
- To avoid a separate library for state management
At times in a small to medium size applications it is needed to share state across components. By using another library for it can impact the performance of your application as each state change triggers a full re-rendering of connected components.
In such scenarios going for Context API provided by react is a good choice.
How to use Context API?
Suppose we want to create an application where we want to know the user details across different components (Profile and Dashboard) within the scope.
-
Create a separate folder named contexts like we do it for custom hooks
-
Inside this folder create a file named UserContext.js
-
Create a context
import createContext from react and create one like below
import React, { createContext, useState } from 'react'; export const UserContext = createContext();
-
Create a provider componentin the same file. From here we will make the necessary state available to make available to other components using the Provider. In our case, we have a user state and a login and logout method that update the user state.
export const UserProvider = ({ children }) => { // default state const [user, setUser] = useState({ name: "Rupali", email: "xyz@abc.com" }); const login = (userData) => { setUser(userData); }; const logout = () => { setUser(null); }; return ( <UserContext.Provider value={{ user, login, logout }}> {children} </UserContext.Provider> ); };
-
Wrap the components with the provider
// App.js import Dashboard from './Dashboard.js'; import Profile from './Profile.js'; const App = () => ( <UserProvider> <Profile /> <Dashboard /> </UserProvider> );
-
Consume the Context Now that we have our context created and provided at root level, let's consume it inside the Dashboard and Profile like this by using useUserContext
// Dashboard.js import { useContext } from 'react'; import { UserContext } from '../contexts/UserContext.js'; const Dashboard = () => { const { user } = useContext(UserContext); return ( <div> <h2>Dashboard</h2> {user && <p>Welcome to the dashboard, {user?.name}!</p>} </div> ); };
// Profile .js import { useContext } from 'react'; import { UserContext } from '../contexts/UserContext.js'; const Profile = () => { const { user, logout } = useContext(UserContext); return ( <div> {user ? ( <> <h2>Welcome, {user.name}!</h2> <p>Email: {user.email}</p> <button onClick={logout}>Logout</button> </> ) : ( <p>Please log in </p> )} </div> ); };
Bonus!!😎 You can see this complete working code in the codesandbox below:
example in codesandbox
Conclusion
While Redux is efficient, but it is worth reevalutating your application needs and do you still really need Redux.