Stop using Redux for state management | Alternatives to redux
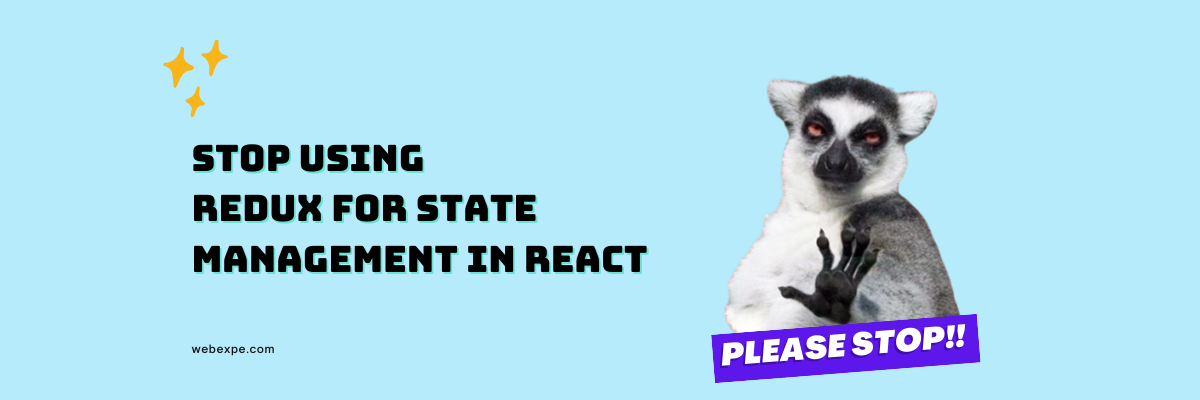
Redux has gained immense popularity as a state management library and has become a staple in every project. However, there are times where developers tend to overuse Redux, even when it might not be necessary.
In this article, we will see what can be used instead of redux for different state mangement scenarios. So let's get started!
But before we get into when not to use Redux let's have a look at why Redux is so famous!
Benefits of Redux
-
Centralized State:
Redux stores the application state in a single store, making it easy to access and update from any component within the application.
-
Predictable Updates:
Due to the use of pure functions called reducers to update the state, it is always easy to predict which inputs can give you what results.
-
Easy config:
It has a very easy setup that is explained on its official website.
-
Easy to debug:
With the use of redux dev tools it becomes easy for debugging complex states.
All these benefits make Redux an attractive choice for state management, but a developer needs to first understand whether Redux is truly necessary for their scenario. Let's see some of the alternatives below.
When can Redux be avoided ?
-
Local Component State using a useState hook
There are so many times when the state required by a component is local and doesn't need to be shared across the application. In such scenarios it is best to use local state using a useState hook.
Consider the below example, where we have an input that takes user's phone number and sends this number to an API on submit. As this number is no longer required to be shared between other components local state is the best choice here.
import React, { useState } from 'react'; const Form = () => { const [phoneNumber, setPhoneNumber] = useState(''); return ( <> <input type="number" value={phoneNumber} onChange={(e)=> setPhoneNumber(e.target.value)} /> <p>Entered value: {name}</p> <button type="button" onClick={()=> {/* Some API call on the button */} } > Submit </button> </> ) }
-
Context API for small to medium sized Applications
At times in a small to medium size applications it is needed to share state across components. In such scenarios we can still avoid using Redux and go for Context API provided by react.
To read more on context API, read my previous article - what is Context API ?
-
Minimal Shared State by passing props and state down
There are times when state and props defined in the parent component are only needed by the children. The best way to handle such scenarios is to pass state and props down to children.
Consider below example where we passed the state todos and removeTodo function that updates the Todos to TodoList child component.
// PARENT COMPONENT const App = () => { const [todos, setTodos] = useState([]); const removeTodo = (index) => { const updatedTodos = todos.filter((item, i) => i !== index); setTodos(updatedTodos); }; return ( <div> <h2>Todo List</h2> <TodoList todos={todos} removeTodo={removeTodo} /> </div> ); }; // CHILD COMPONENT const TodoList = ({ todos, removeTodo }) => { return ( <ul> {todos.map((todo, index) => ( <TodoItem key={index} todo={todo} removeTodo={() => removeTodo(index)} /> ))} </ul> ); };
Conclusion
While Redux is efficient, but it is worth reevalutating your application needs and do you still really need Redux.